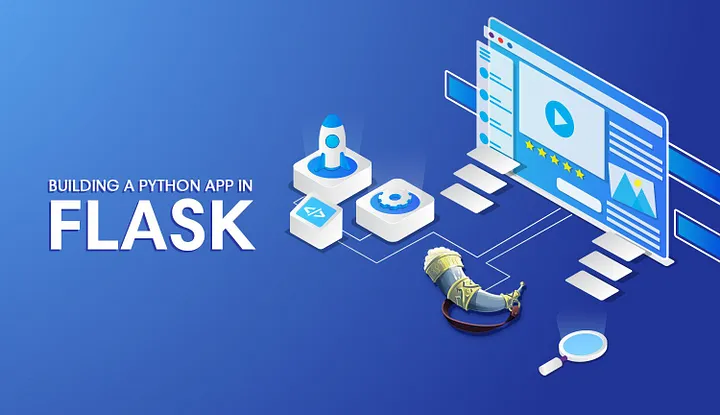
Building Robust REST APIs with Python Flask: A Guide for Developers
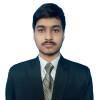
In web development, creating robust and efficient RESTful APIs is essential for building scalable and interoperable applications. With the rise in demand for dynamic web services, developers are turning to lightweight frameworks like Flask to streamline the development process and deliver powerful APIs with minimal overhead. In this guide, we’ll explore how to leverage Python Flask to build RESTful APIs that meet the needs of modern web applications.
Introduction to Flask
Flask is a micro-framework for Python that provides a simple yet powerful foundation for building web applications and APIs. Known for its minimalistic design and flexibility, Flask offers developers the freedom to structure their applications according to their specific requirements without imposing unnecessary constraints. With Flask, you can quickly set up routes, handle requests and responses, and integrate with third-party libraries to extend functionality.
Setting Up Your Development Environment
Before diving into API development with Flask, it’s essential to set up your development environment. Start by creating a new Python virtual environment to isolate dependencies for your project. Once your virtual environment is activated, use pip to install Flask and any additional libraries you may need for your project, such as Flask-RESTful for building RESTful APIs.
$ python -m venv venv
$ source venv/bin/activate
(venv) $ pip install Flask Flask-RESTful
Creating Your First Flask API
With your development environment configured, you’re ready to start building your first Flask API. Begin by creating a new Python script for your application, such as app.py. In this script, import the necessary modules from Flask and Flask-RESTful, and define your API endpoints using Flask’s routing system.
from flask import Flask
from flask_restful import Api, Resource
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'message': 'Hello, World!'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
In this example, we’ve defined a simple API endpoint that responds with a JSON message containing “Hello, World!” when accessed via a GET request to the root URL (/).
Handling Request Data
As your API becomes more complex, you’ll often need to handle request data such as query parameters, request headers, and request bodies. Flask provides convenient methods for accessing this data within your route handlers.
from flask import request
class User(Resource):
def get(self, user_id):
# Retrieve user data from database based on user_id
return {'user_id': user_id, 'name': 'John Doe'}
def post(self):
data = request.get_json()
# Process and store user data from request body
return {'message': 'User created successfully', 'data': data}, 201
Error Handling and Responses
In a production API, it’s essential to handle errors gracefully and provide meaningful responses to clients. Flask allows you to define custom error handlers and return appropriate HTTP status codes and error messages.
from flask import jsonify
@app.errorhandler(404)
def not_found(error):
return jsonify({'error': 'Not found'}), 404
Testing Your Flask API
Testing is a crucial aspect of API development to ensure reliability and consistency. Flask provides built-in support for testing using the Werkzeug test client, allowing you to simulate HTTP requests and assert expected responses.
import unittest
class TestAPI(unittest.TestCase):
def setUp(self):
self.app = app.test_client()
def test_hello_world(self):
response = self.app.get('/')
self.assertEqual(response.status_code, 200)
self.assertEqual(response.json['message'], 'Hello, World!')
if __name__ == '__main__':
unittest.main()
Conclusion
With its simplicity, flexibility, and a robust ecosystem of extensions, Flask is an excellent choice for building RESTful APIs in Python. By following the principles outlined in this guide, you can leverage Flask to create scalable, interoperable APIs that power modern web applications with ease. Whether you’re a seasoned developer or just getting started with web development, Flask empowers you to bring your ideas to life and deliver exceptional user experiences through well-designed APIs. Happy coding!